Hi all,
I'd thought I'd mention that I'm working on a project (part fun/learning/useful) called
Pas6502 which will compile a dialect of Pascal into 6502 assembly code (using Kick Assembler macros and asm code). I will be starting with the C64.
I know there have been others, but I wanted to do this myself too

I have made a start where I can parse expressions using standard Pascal syntax, or expressions including mixed maths & boolean logic for tricky equations like the
Dirac delta function (one line):
Code:
a := -(x=0)
or the
absolute value function (definitely tricky code!):
Code:
x := x*(1+2*(x<0))
Which currently produces this code:
Code:
main:
:loadIntRegMem(x,0)
:loadIntRegIm(1,1)
:loadIntRegIm(2,2)
:loadIntRegMem(x,3)
:loadIntRegIm(0,4)
:cmpIntRegLss(3,4,3)
:mulIntReg(2,3,2)
:addIntReg(1,2,1)
:mulIntReg(0,1,0)
:storeIntRegMem(0,x)
rts
I will be adding in expression simplification too which will include power of 2 div/mull -> shifts/shifts+adds.
Internally, I will be representing
False = 0 and
True = -1, but any value <> 0 will also equate to True so I will be able to things like;
Code:
if SomeNumericVar then
// do something if SomeNumericVar <> 0
...
I am also posting on twitter:
https://twitter.com/SyntaxErrorSoft/status/1063554525586321409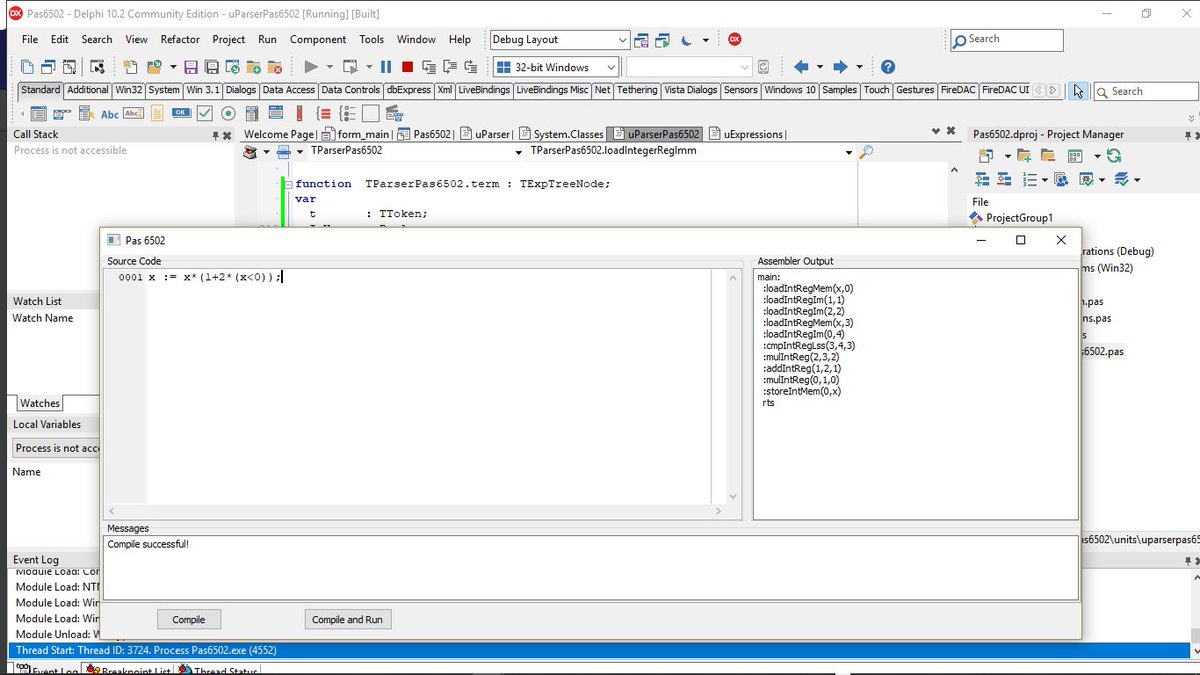
cheers,
Paul